Given an array of integers arr[] of size N and an integer, the task is to rotate the array elements to the left by d positions.
Examples:
Input:
arr[] = {1, 2, 3, 4, 5, 6, 7}, d = 2
Output: 3 4 5 6 7 1 2
Input: arr[] = {3, 4, 5, 6, 7, 1, 2}, d=2
Output: 5 6 7 1 2 3 4
Approach 1 (Using temp array): This problem can be solved using the below idea:
After rotating d positions to the left, the first d elements become the last d elements of the array
- First store the elements from index d to N-1 into the temp array.
- Then store the first d elements of the original array into the temp array.
- Copy back the elements of the temp array into the original array
Illustration:
Suppose the give array is arr[] = [1, 2, 3, 4, 5, 6, 7], d = 2.
First Step:
=> Store the elements from 2nd index to the last.
=> temp[] = [3, 4, 5, 6, 7]
Second Step:
=> Now store the first 2 elements into the temp[] array.
=> temp[] = [3, 4, 5, 6, 7, 1, 2]
Third Steps:
=> Copy the elements of the temp[] array into the original array.
=> arr[] = temp[] So arr[] = [3, 4, 5, 6, 7, 1, 2]
Follow the steps below to solve the given problem.
- Initialize a temporary array(temp[n]) of length same as the original array
- Initialize an integer(k) to keep a track of the current index
- Store the elements from the position d to n-1 in the temporary array
- Now, store 0 to d-1 elements of the original array in the temporary array
- Lastly, copy back the temporary array to the original array
Below is the implementation of the above approach :
C++
#include <bits/stdc++.h>
using namespace std;
void Rotate( int arr[], int d, int n)
{
int temp[n];
int k = 0;
for ( int i = d; i < n; i++) {
temp[k] = arr[i];
k++;
}
for ( int i = 0; i < d; i++) {
temp[k] = arr[i];
k++;
}
for ( int i = 0; i < n; i++) {
arr[i] = temp[i];
}
}
void PrintTheArray( int arr[], int n)
{
for ( int i = 0; i < n; i++) {
cout << arr[i] << " " ;
}
}
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 6, 7 };
int N = sizeof (arr) / sizeof (arr[0]);
int d = 2;
Rotate(arr, d, N);
PrintTheArray(arr, N);
return 0;
}
|
Java
import java.io.*;
class GFG {
static void Rotate( int arr[], int d, int n)
{
int temp[] = new int [n];
int k = 0 ;
for ( int i = d; i < n; i++) {
temp[k] = arr[i];
k++;
}
for ( int i = 0 ; i < d; i++) {
temp[k] = arr[i];
k++;
}
for ( int i = 0 ; i < n; i++) {
arr[i] = temp[i];
}
}
static void PrintTheArray( int arr[], int n)
{
for ( int i = 0 ; i < n; i++) {
System.out.print(arr[i]+ " " );
}
}
public static void main (String[] args) {
int arr[] = { 1 , 2 , 3 , 4 , 5 , 6 , 7 };
int N = arr.length;
int d = 2 ;
Rotate(arr, d, N);
PrintTheArray(arr, N);
}
}
|
Python3
def rotate(L, d, n):
k = L.index(d)
new_lis = []
new_lis = L[k + 1 :] + L[ 0 :k + 1 ]
return new_lis
if __name__ = = '__main__' :
arr = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 ]
d = 2
N = len (arr)
arr = rotate(arr, d, N)
for i in arr:
print (i, end = " " )
|
C#
using System;
public class GFG
{
public static void Rotate( int [] arr, int d, int n)
{
int [] temp = new int [n];
var k = 0;
for ( int i = d; i < n; i++)
{
temp[k] = arr[i];
k++;
}
for ( int i = 0; i < d; i++)
{
temp[k] = arr[i];
k++;
}
for ( int i = 0; i < n; i++)
{
arr[i] = temp[i];
}
}
public static void PrintTheArray( int [] arr, int n)
{
for ( int i = 0; i < n; i++)
{
Console.Write(arr[i].ToString() + " " );
}
}
public static void Main(String[] args)
{
int [] arr = {1, 2, 3, 4, 5, 6, 7};
var N = arr.Length;
var d = 2;
GFG.Rotate(arr, d, N);
GFG.PrintTheArray(arr, N);
}
}
|
Javascript
function Rotate_and_Print(arr,d,n)
{
var temp= new Array(n);
let k = 0;
for (let i = d; i < n; i++) {
temp[k] = arr[i];
k++;
}
for (let i = 0; i < d; i++) {
temp[k] = arr[i];
k++;
}
for (let i = 0; i < n; i++) {
console.log(temp[i]+ " " );
}
}
let arr = [ 1, 2, 3, 4, 5, 6, 7 ];
let n = arr.length;
let d = 2;
Rotate_and_Print(arr, d, n);
|
Time complexity: O(N)
Auxiliary Space: O(N)
Approach 2 (Rotate one by one): This problem can be solved using the below idea:
- At each iteration, shift the elements by one position to the left circularly (i.e., first element becomes the last).
- Perform this operation d times to rotate the elements to the left by d position.
Illustration:
Let us take arr[] = [1, 2, 3, 4, 5, 6, 7], d = 2.
First Step:
=> Rotate to left by one position.
=> arr[] = {2, 3, 4, 5, 6, 7, 1}
Second Step:
=> Rotate again to left by one position
=> arr[] = {3, 4, 5, 6, 7, 1, 2}
Rotation is done by 2 times.
So the array becomes arr[] = {3, 4, 5, 6, 7, 1, 2}
Follow the steps below to solve the given problem.
- Rotate the array to left by one position. For that do the following:
- Store the first element of the array in a temporary variable.
- Shift the rest of the elements in the original array by one place.
- Update the last index of the array with the temporary variable.
- Repeat the above steps for the number of left rotations required.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void Rotate( int arr[], int d, int n)
{
int p = 1;
while (p <= d) {
int last = arr[0];
for ( int i = 0; i < n - 1; i++) {
arr[i] = arr[i + 1];
}
arr[n - 1] = last;
p++;
}
}
void printArray( int arr[], int size)
{
for ( int i = 0; i < size; i++)
cout << arr[i] << " " ;
}
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 6, 7 };
int N = sizeof (arr) / sizeof (arr[0]);
int d = 2;
Rotate(arr, d, N);
printArray(arr, N);
return 0;
}
|
Java
import java.io.*;
class GFG {
public static void rotate( int arr[], int d, int n)
{
int p = 1 ;
while (p <= d) {
int last = arr[ 0 ];
for ( int i = 0 ; i < n - 1 ; i++) {
arr[i] = arr[i + 1 ];
}
arr[n - 1 ] = last;
p++;
}
for ( int i = 0 ; i < n; i++) {
System.out.print(arr[i] + " " );
}
}
public static void main(String[] args)
{
int arr[] = { 1 , 2 , 3 , 4 , 5 , 6 , 7 };
int N = arr.length;
int d = 2 ;
rotate(arr, d, N);
}
}
|
Python3
def Rotate(arr, d, n):
p = 1
while (p < = d):
last = arr[ 0 ]
for i in range (n - 1 ):
arr[i] = arr[i + 1 ]
arr[n - 1 ] = last
p = p + 1
def printArray(arr, size):
for i in range (size):
print (arr[i] ,end = " " )
arr = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 ]
N = len (arr)
d = 2
Rotate(arr, d, N)
printArray(arr, N)
|
C#
using System;
public class GFG
{
public static void rotate( int [] arr, int d, int n)
{
var p = 1;
while (p <= d)
{
var last = arr[0];
for ( int i = 0; i < n - 1; i++)
{
arr[i] = arr[i + 1];
}
arr[n - 1] = last;
p++;
}
for ( int i = 0; i < n; i++)
{
Console.Write(arr[i].ToString() + " " );
}
}
public static void Main(String[] args)
{
int [] arr = {1, 2, 3, 4, 5, 6, 7};
var N = arr.Length;
var d = 2;
GFG.rotate(arr, d, N);
}
}
|
Javascript
function printArray(arr,n,d)
{
let p = 1;
while (p <= d) {
let last = arr[0];
for (let i = 0; i < n - 1; i++) {
arr[i] = arr[i + 1];
}
arr[n - 1] = last;
p++;
}
for (let i = 0; i < n; i++) {
console.log(arr[i] + " " );
}
}
let arr = [ 1, 2, 3, 4, 5, 6, 7 ];
let n = arr.length;
let d=2;
printArray(arr, n,d);
|
Time Complexity: O(N * d)
Auxiliary Space: O(1)
Approach 3 (A Juggling Algorithm): This is an extension of method 2.
Instead of moving one by one, divide the array into different sets where the number of sets is equal to the GCD of N and d (say X. So the elements which are X distance apart are part of a set) and rotate the elements within sets by 1 position to the left.
- Calculate the GCD between the length and the distance to be moved.
- The elements are only shifted within the sets.
- We start with temp = arr[0] and keep moving arr[I+d] to arr[I] and finally store temp at the right place.
Follow the below illustration for a better understanding
Illustration:
Each steps looks like following:
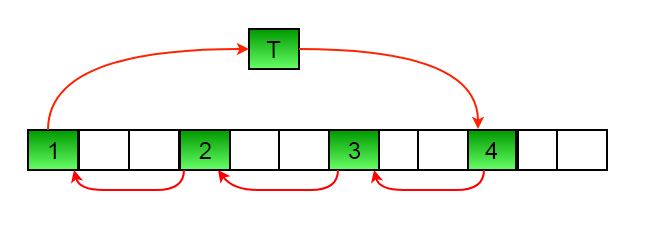
Let arr[] = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14} and d = 10
First step:
=> First set is {0, 5, 10}.
=> Rotate this set by d position in cyclic order
=> arr[0] = arr[0+10]
=> arr[10] = arr[(10+10)%15]
=> arr[5] = arr[0]
=> This set becomes {10,0,5}
=> Array arr[] = {10, 1, 2, 3, 4, 0, 6, 7, 8, 9, 5, 11, 12, 13, 14}
Second step:
=> Second set is {1, 6, 11}.
=> Rotate this set by d position in cyclic order.
=> This set becomes {11, 1, 6}
=> Array arr[] = {10, 11, 2, 3, 4, 0, 1, 7, 8, 9, 5, 6, 12, 13, 14}
Third step:
=> Second set is {2, 7, 12}.
=> Rotate this set by d position in cyclic order.
=> This set becomes {12, 2, 7}
=> Array arr[] = {10, 11, 12, 3, 4, 0, 1, 2, 8, 9, 5, 6, 7, 13, 14}
Fourth step:
=> Second set is {3, 8, 13}.
=> Rotate this set by d position in cyclic order.
=> This set becomes {13, 3, 8}
=> Array arr[] = {10, 11, 12, 13, 4, 0, 1, 2, 3, 9, 5, 6, 7, 8, 14}
Fifth step:
=> Second set is {4, 9, 14}.
=> Rotate this set by d position in cyclic order.
=> This set becomes {14, 4, 9}
=> Array arr[] = {10, 11, 12, 13, 14, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9}
Follow the steps below to solve the given problem.
- Perform d%n in order to keep the value of d within the range of the array where d is the number of times the array is rotated and N is the size of the array.
- Calculate the GCD(N, d) to divide the array into sets.
- Run a for loop from 0 to the value obtained from GCD.
- Store the value of arr[i] in a temporary variable (the value of i denotes the set number).
- Run a while loop to update the values according to the set.
- After exiting the while loop assign the value of arr[j] as the value of the temporary variable (the value of j denotes the last element of the ith set).
Below is the implementation of the above approach :
C++
#include <bits/stdc++.h>
using namespace std;
int gcd( int a, int b)
{
if (b == 0)
return a;
else
return gcd(b, a % b);
}
void leftRotate( int arr[], int d, int n)
{
d = d % n;
int g_c_d = gcd(d, n);
for ( int i = 0; i < g_c_d; i++) {
int temp = arr[i];
int j = i;
while (1) {
int k = j + d;
if (k >= n)
k = k - n;
if (k == i)
break ;
arr[j] = arr[k];
j = k;
}
arr[j] = temp;
}
}
void printArray( int arr[], int size)
{
for ( int i = 0; i < size; i++)
cout << arr[i] << " " ;
}
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 6, 7 };
int n = sizeof (arr) / sizeof (arr[0]);
leftRotate(arr, 2, n);
printArray(arr, n);
return 0;
}
|
C
#include <stdio.h>
void printArray( int arr[], int size);
int gcd( int a, int b);
void leftRotate( int arr[], int d, int n)
{
int i, j, k, temp;
d = d % n;
int g_c_d = gcd(d, n);
for (i = 0; i < g_c_d; i++) {
temp = arr[i];
j = i;
while (1) {
k = j + d;
if (k >= n)
k = k - n;
if (k == i)
break ;
arr[j] = arr[k];
j = k;
}
arr[j] = temp;
}
}
void printArray( int arr[], int n)
{
int i;
for (i = 0; i < n; i++)
printf ( "%d " , arr[i]);
}
int gcd( int a, int b)
{
if (b == 0)
return a;
else
return gcd(b, a % b);
}
int main()
{
int arr[] = { 1, 2, 3, 4, 5, 6, 7 };
leftRotate(arr, 2, 7);
printArray(arr, 7);
getchar ();
return 0;
}
|
Java
import java.io.*;
class RotateArray {
void leftRotate( int arr[], int d, int n)
{
d = d % n;
int i, j, k, temp;
int g_c_d = gcd(d, n);
for (i = 0 ; i < g_c_d; i++) {
temp = arr[i];
j = i;
while ( true ) {
k = j + d;
if (k >= n)
k = k - n;
if (k == i)
break ;
arr[j] = arr[k];
j = k;
}
arr[j] = temp;
}
}
void printArray( int arr[], int size)
{
int i;
for (i = 0 ; i < size; i++)
System.out.print(arr[i] + " " );
}
int gcd( int a, int b)
{
if (b == 0 )
return a;
else
return gcd(b, a % b);
}
public static void main(String[] args)
{
RotateArray rotate = new RotateArray();
int arr[] = { 1 , 2 , 3 , 4 , 5 , 6 , 7 };
rotate.leftRotate(arr, 2 , 7 );
rotate.printArray(arr, 7 );
}
}
|
Python3
def leftRotate(arr, d, n):
d = d % n
g_c_d = gcd(d, n)
for i in range (g_c_d):
temp = arr[i]
j = i
while 1 :
k = j + d
if k > = n:
k = k - n
if k = = i:
break
arr[j] = arr[k]
j = k
arr[j] = temp
def printArray(arr, size):
for i in range (size):
print ( "% d" % arr[i], end = " " )
def gcd(a, b):
if b = = 0 :
return a
else :
return gcd(b, a % b)
arr = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 ]
n = len (arr)
d = 2
leftRotate(arr, d, n)
printArray(arr, n)
|
C#
using System;
class GFG {
static void leftRotate( int [] arr, int d, int n)
{
int i, j, k, temp;
d = d % n;
int g_c_d = gcd(d, n);
for (i = 0; i < g_c_d; i++) {
temp = arr[i];
j = i;
while ( true ) {
k = j + d;
if (k >= n)
k = k - n;
if (k == i)
break ;
arr[j] = arr[k];
j = k;
}
arr[j] = temp;
}
}
static void printArray( int [] arr, int size)
{
for ( int i = 0; i < size; i++)
Console.Write(arr[i] + " " );
}
static int gcd( int a, int b)
{
if (b == 0)
return a;
else
return gcd(b, a % b);
}
public static void Main()
{
int [] arr = { 1, 2, 3, 4, 5, 6, 7 };
leftRotate(arr, 2, 7);
printArray(arr, 7);
}
}
|
Javascript
<script>
function gcd( a, b){
if (b == 0)
return a;
else
return gcd(b, a % b);
}
function leftRotate(arr, d, n){
d = d % n;
let g_c_d = gcd(d, n);
for (let i = 0; i < g_c_d; i++) {
let temp = arr[i];
let j = i;
while (1) {
let k = j + d;
if (k >= n)
k = k - n;
if (k == i)
break ;
arr[j] = arr[k];
j = k;
}
arr[j] = temp;
}
}
function printArray(arr, size){
for (let i = 0; i < size; i++)
document.write(arr[i] + " " );
}
let arr = [ 1, 2, 3, 4, 5, 6, 7 ];
let n = arr.length;
leftRotate(arr, 2, n);
printArray(arr, n);
</script>
|
Time complexity : O(N)
Auxiliary Space : O(1)
Please see the following posts for other methods of array rotation:
Block swap algorithm for array rotation
Reversal algorithm for array rotation
Please write comments if you find any bugs in the above programs/algorithms.
Feeling lost in the world of random DSA topics, wasting time without progress? It's time for a change! Join our DSA course, where we'll guide you on an exciting journey to master DSA efficiently and on schedule.
Ready to dive in? Explore our Free Demo Content and join our DSA course, trusted by over 100,000 geeks!