Given a postfix expression, the task is to evaluate the postfix expression.
Postfix expression: The expression of the form “a b operator” (ab+) i.e., when a pair of operands is followed by an operator.
Examples:
Input: str = “2 3 1 * + 9 -“
Output: -4
Explanation: If the expression is converted into an infix expression, it will be 2 + (3 * 1) – 9 = 5 – 9 = -4.
Input: str = “100 200 + 2 / 5 * 7 +”
Output: 757
Evaluation of Postfix Expression using Stack:
To evaluate a postfix expression we can use a stack.
Iterate the expression from left to right and keep on storing the operands into a stack. Once an operator is received, pop the two topmost elements and evaluate them and push the result in the stack again.
Illustration:
Follow the below illustration for a better understanding:
Consider the expression: exp = “2 3 1 * + 9 -“
- Scan 2, it’s a number, So push it into stack. Stack contains ‘2’.
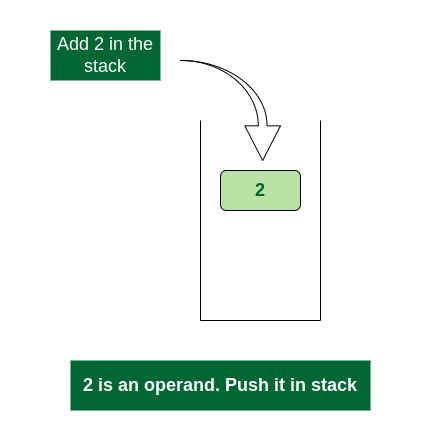
Push 2 into stack
- Scan 3, again a number, push it to stack, stack now contains ‘2 3’ (from bottom to top)
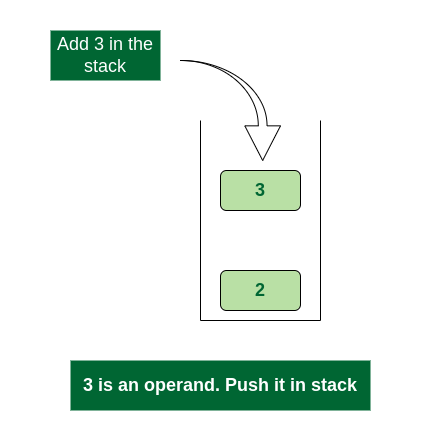
Push 3 into stack
- Scan 1, again a number, push it to stack, stack now contains ‘2 3 1’
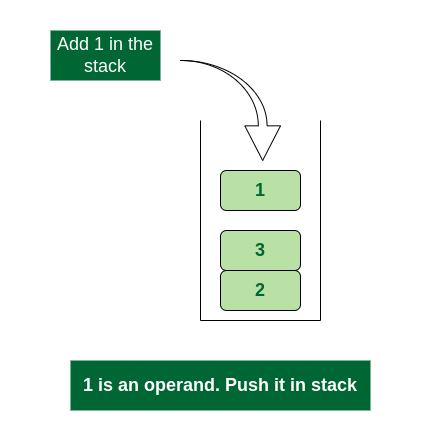
Push 1 into stack
- Scan *, it’s an operator. Pop two operands from stack, apply the * operator on operands. We get 3*1 which results in 3. We push the result 3 to stack. The stack now becomes ‘2 3’.
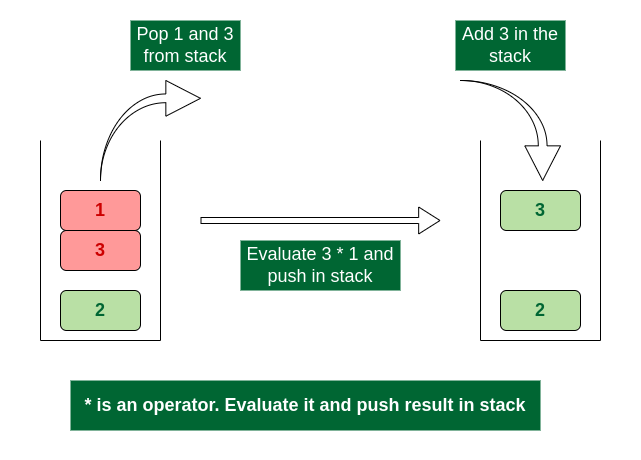
Evaluate * operator and push result in stack
- Scan +, it’s an operator. Pop two operands from stack, apply the + operator on operands. We get 3 + 2 which results in 5. We push the result 5 to stack. The stack now becomes ‘5’.
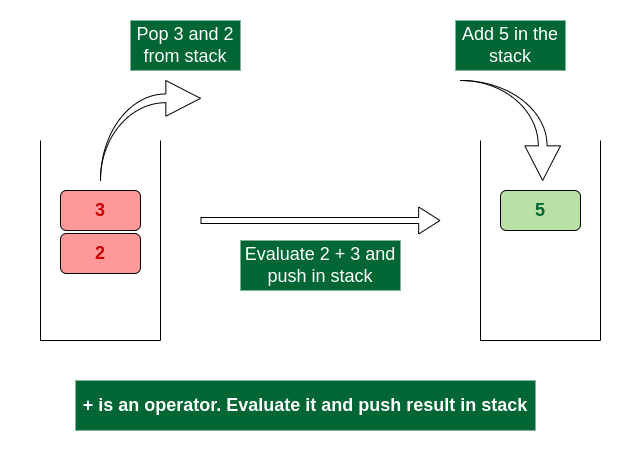
Evaluate + operator and push result in stack
- Scan 9, it’s a number. So we push it to the stack. The stack now becomes ‘5 9’.
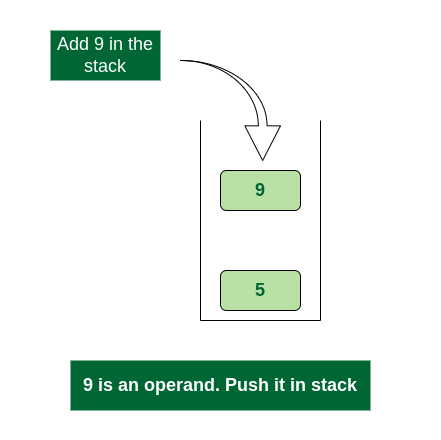
Push 9 into stack
- Scan -, it’s an operator, pop two operands from stack, apply the – operator on operands, we get 5 – 9 which results in -4. We push the result -4 to the stack. The stack now becomes ‘-4’.
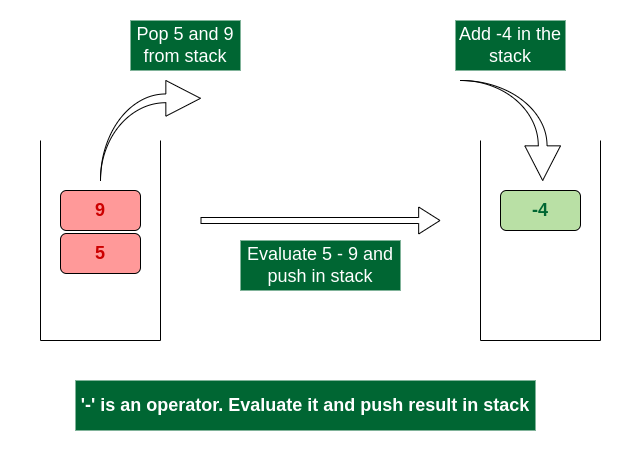
Evaluate ‘-‘ operator and push result in stack
- There are no more elements to scan, we return the top element from the stack (which is the only element left in a stack).
So the result becomes -4.
Follow the steps mentioned below to evaluate postfix expression using stack:
- Create a stack to store operands (or values).
- Scan the given expression from left to right and do the following for every scanned element.
- If the element is a number, push it into the stack.
- If the element is an operator, pop operands for the operator from the stack. Evaluate the operator and push the result back to the stack.
- When the expression is ended, the number in the stack is the final answer.
Below is the implementation of the above approach:
C
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Stack {
int top;
unsigned capacity;
int * array;
};
struct Stack* createStack(unsigned capacity)
{
struct Stack* stack
= ( struct Stack*) malloc ( sizeof ( struct Stack));
if (!stack)
return NULL;
stack->top = -1;
stack->capacity = capacity;
stack->array
= ( int *) malloc (stack->capacity * sizeof ( int ));
if (!stack->array)
return NULL;
return stack;
}
int isEmpty( struct Stack* stack)
{
return stack->top == -1;
}
char peek( struct Stack* stack)
{
return stack->array[stack->top];
}
char pop( struct Stack* stack)
{
if (!isEmpty(stack))
return stack->array[stack->top--];
return '$' ;
}
void push( struct Stack* stack, char op)
{
stack->array[++stack->top] = op;
}
int evaluatePostfix( char * exp )
{
struct Stack* stack = createStack( strlen ( exp ));
int i;
if (!stack)
return -1;
for (i = 0; exp [i]; ++i) {
if ( isdigit ( exp [i]))
push(stack, exp [i] - '0' );
else {
int val1 = pop(stack);
int val2 = pop(stack);
switch ( exp [i]) {
case '+' :
push(stack, val2 + val1);
break ;
case '-' :
push(stack, val2 - val1);
break ;
case '*' :
push(stack, val2 * val1);
break ;
case '/' :
push(stack, val2 / val1);
break ;
}
}
}
return pop(stack);
}
int main()
{
char exp [] = "231*+9-" ;
printf ( "postfix evaluation: %d" , evaluatePostfix( exp ));
return 0;
}
|
C++
#include <bits/stdc++.h>
using namespace std;
int evaluatePostfix(string exp )
{
stack< int > st;
for ( int i = 0; i < exp .size(); ++i) {
if ( isdigit ( exp [i]))
st.push( exp [i] - '0' );
else {
int val1 = st.top();
st.pop();
int val2 = st.top();
st.pop();
switch ( exp [i]) {
case '+' :
st.push(val2 + val1);
break ;
case '-' :
st.push(val2 - val1);
break ;
case '*' :
st.push(val2 * val1);
break ;
case '/' :
st.push(val2 / val1);
break ;
}
}
}
return st.top();
}
int main()
{
string exp = "231*+9-" ;
cout << "postfix evaluation: " << evaluatePostfix( exp );
return 0;
}
|
Java
import java.util.Stack;
public class Test {
static int evaluatePostfix(String exp)
{
Stack<Integer> stack = new Stack<>();
for ( int i = 0 ; i < exp.length(); i++) {
char c = exp.charAt(i);
if (Character.isDigit(c))
stack.push(c - '0' );
else {
int val1 = stack.pop();
int val2 = stack.pop();
switch (c) {
case '+' :
stack.push(val2 + val1);
break ;
case '-' :
stack.push(val2 - val1);
break ;
case '/' :
stack.push(val2 / val1);
break ;
case '*' :
stack.push(val2 * val1);
break ;
}
}
}
return stack.pop();
}
public static void main(String[] args)
{
String exp = "231*+9-" ;
System.out.println( "postfix evaluation: "
+ evaluatePostfix(exp));
}
}
|
Python3
class Evaluate:
def __init__( self , capacity):
self .top = - 1
self .capacity = capacity
self .array = []
def isEmpty( self ):
return True if self .top = = - 1 else False
def peek( self ):
return self .array[ - 1 ]
def pop( self ):
if not self .isEmpty():
self .top - = 1
return self .array.pop()
else :
return "$"
def push( self , op):
self .top + = 1
self .array.append(op)
def evaluatePostfix( self , exp):
for i in exp:
if i.isdigit():
self .push(i)
else :
val1 = self .pop()
val2 = self .pop()
self .push( str ( eval (val2 + i + val1)))
return int ( self .pop())
if __name__ = = '__main__' :
exp = "231*+9-"
obj = Evaluate( len (exp))
print ( "postfix evaluation: %d" % (obj.evaluatePostfix(exp)))
|
C#
using System;
using System.Collections;
namespace GFG {
class Geek {
static void Main()
{
Geek e = new Geek();
e.v = ( "231*+9-" );
e.expression();
Console.WriteLine( "postfix evaluation: "
+ e.answer);
Console.Read();
}
public string v;
public string answer;
Stack i = new Stack();
public void expression()
{
int a, b, ans;
for ( int j = 0; j < v.Length; j++)
{
String c = v.Substring(j, 1);
if (c.Equals( "*" )) {
String sa = (String)i.Pop();
String sb = (String)i.Pop();
a = Convert.ToInt32(sb);
b = Convert.ToInt32(sa);
ans = a * b;
i.Push(ans.ToString());
}
else if (c.Equals( "/" )) {
String sa = (String)i.Pop();
String sb = (String)i.Pop();
a = Convert.ToInt32(sb);
b = Convert.ToInt32(sa);
ans = a / b;
i.Push(ans.ToString());
}
else if (c.Equals( "+" )) {
String sa = (String)i.Pop();
String sb = (String)i.Pop();
a = Convert.ToInt32(sb);
b = Convert.ToInt32(sa);
ans = a + b;
i.Push(ans.ToString());
}
else if (c.Equals( "-" )) {
String sa = (String)i.Pop();
String sb = (String)i.Pop();
a = Convert.ToInt32(sb);
b = Convert.ToInt32(sa);
ans = a - b;
i.Push(ans.ToString());
}
else {
i.Push(v.Substring(j, 1));
}
}
answer = (String)i.Pop();
}
}
}
|
Javascript
<script>
function evaluatePostfix(exp)
{
let stack=[];
for (let i=0;i<exp.length;i++)
{
let c=exp[i];
if (! isNaN( parseInt(c) ))
stack.push(c.charCodeAt(0) - '0' .charCodeAt(0));
else
{
let val1 = stack.pop();
let val2 = stack.pop();
switch (c)
{
case '+' :
stack.push(val2+val1);
break ;
case '-' :
stack.push(val2- val1);
break ;
case '/' :
stack.push(val2/val1);
break ;
case '*' :
stack.push(val2*val1);
break ;
}
}
}
return stack.pop();
}
let exp= "231*+9-" ;
document.write( "postfix evaluation: " +evaluatePostfix(exp));
</script>
|
Output
postfix evaluation: -4
Time Complexity: O(N)
Auxiliary Space: O(N)
There are the following limitations of the above implementation.
- It supports only 4 binary operators ‘+’, ‘*’, ‘-‘ and ‘/’. It can be extended for more operators by adding more switch cases.
- The allowed operands are only single-digit operands.
Postfix evaluation for multi-digit numbers:
The above program can be extended for multiple digits by adding a separator-like space between all elements (operators and operands) of the given expression.
Below given is the extended program which allows operands to have multiple digits.
C
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Stack {
int top;
unsigned capacity;
int * array;
};
struct Stack* createStack(unsigned capacity)
{
struct Stack* stack
= ( struct Stack*) malloc ( sizeof ( struct Stack));
if (!stack)
return NULL;
stack->top = -1;
stack->capacity = capacity;
stack->array
= ( int *) malloc (stack->capacity * sizeof ( int ));
if (!stack->array)
return NULL;
return stack;
}
int isEmpty( struct Stack* stack)
{
return stack->top == -1;
}
int peek( struct Stack* stack)
{
return stack->array[stack->top];
}
int pop( struct Stack* stack)
{
if (!isEmpty(stack))
return stack->array[stack->top--];
return '$' ;
}
void push( struct Stack* stack, int op)
{
stack->array[++stack->top] = op;
}
int evaluatePostfix( char * exp )
{
struct Stack* stack = createStack( strlen ( exp ));
int i;
if (!stack)
return -1;
for (i = 0; exp [i]; ++i) {
if ( exp [i] == ' ' )
continue ;
else if ( isdigit ( exp [i])) {
int num = 0;
while ( isdigit ( exp [i])) {
num = num * 10 + ( int )( exp [i] - '0' );
i++;
}
i--;
push(stack, num);
}
else {
int val1 = pop(stack);
int val2 = pop(stack);
switch ( exp [i]) {
case '+' :
push(stack, val2 + val1);
break ;
case '-' :
push(stack, val2 - val1);
break ;
case '*' :
push(stack, val2 * val1);
break ;
case '/' :
push(stack, val2 / val1);
break ;
}
}
}
return pop(stack);
}
int main()
{
char exp [] = "100 200 + 2 / 5 * 7 +" ;
printf ( "%d" , evaluatePostfix( exp ));
return 0;
}
|
C++14
#include <bits/stdc++.h>
using namespace std;
int evaluatePostfix( char * exp )
{
stack< int > st;
int i;
for (i = 0; exp [i]; ++i) {
if ( exp [i] == ' ' )
continue ;
else if ( isdigit ( exp [i])) {
int num = 0;
while ( isdigit ( exp [i])) {
num = num * 10 + ( int )( exp [i] - '0' );
i++;
}
i--;
st.push(num);
}
else {
int val1 = st.top();
st.pop();
int val2 = st.top();
st.pop();
switch ( exp [i]) {
case '+' :
st.push(val2 + val1);
break ;
case '-' :
st.push(val2 - val1);
break ;
case '*' :
st.push(val2 * val1);
break ;
case '/' :
st.push(val2 / val1);
break ;
}
}
}
return st.top();
}
int main()
{
char exp [] = "100 200 + 2 / 5 * 7 +" ;
cout << evaluatePostfix( exp );
return 0;
}
|
Java
import java.util.Stack;
class Test1 {
static int evaluatePostfix(String exp)
{
Stack<Integer> stack = new Stack<>();
for ( int i = 0 ; i < exp.length(); i++) {
char c = exp.charAt(i);
if (c == ' ' )
continue ;
else if (Character.isDigit(c)) {
int n = 0 ;
while (Character.isDigit(c)) {
n = n * 10 + ( int )(c - '0' );
i++;
c = exp.charAt(i);
}
i--;
stack.push(n);
}
else {
int val1 = stack.pop();
int val2 = stack.pop();
switch (c) {
case '+' :
stack.push(val2 + val1);
break ;
case '-' :
stack.push(val2 - val1);
break ;
case '/' :
stack.push(val2 / val1);
break ;
case '*' :
stack.push(val2 * val1);
break ;
}
}
}
return stack.pop();
}
public static void main(String[] args)
{
String exp = "100 200 + 2 / 5 * 7 +" ;
System.out.println(evaluatePostfix(exp));
}
}
|
Python3
class evalpostfix:
def __init__( self ):
self .stack = []
self .top = - 1
def pop( self ):
if self .top = = - 1 :
return
else :
self .top - = 1
return self .stack.pop()
def push( self , i):
self .top + = 1
self .stack.append(i)
def centralfunc( self , ab):
for i in ab:
try :
self .push( int (i))
except ValueError:
val1 = self .pop()
val2 = self .pop()
if i = = '/' :
self .push(val2 / val1)
else :
switcher = { '+' : val2 + val1, '-' : val2 -
val1, '*' : val2 * val1, '^' : val2 * * val1}
self .push(switcher.get(i))
return int ( self .pop())
if __name__ = = '__main__' :
str = '100 200 + 2 / 5 * 7 +'
strconv = str .split( ' ' )
obj = evalpostfix()
print (obj.centralfunc(strconv))
|
C#
using System;
using System.Collections.Generic;
class GFG {
public static int evaluatePostfix( string exp)
{
Stack< int > stack = new Stack< int >();
for ( int i = 0; i < exp.Length; i++) {
char c = exp[i];
if (c == ' ' ) {
continue ;
}
else if ( char .IsDigit(c)) {
int n = 0;
while ( char .IsDigit(c)) {
n = n * 10 + ( int )(c - '0' );
i++;
c = exp[i];
}
i--;
stack.Push(n);
}
else {
int val1 = stack.Pop();
int val2 = stack.Pop();
switch (c) {
case '+' :
stack.Push(val2 + val1);
break ;
case '-' :
stack.Push(val2 - val1);
break ;
case '/' :
stack.Push(val2 / val1);
break ;
case '*' :
stack.Push(val2 * val1);
break ;
}
}
}
return stack.Pop();
}
public static void Main( string [] args)
{
string exp = "100 200 + 2 / 5 * 7 +" ;
Console.WriteLine(evaluatePostfix(exp));
}
}
|
Javascript
<script>
function evaluatePostfix(exp)
{
let stack = [];
for (let i = 0; i < exp.length; i++)
{
let c = exp[i];
if (c == ' ' )
{
continue ;
}
else if (c >= '0' && c <= '9' )
{
let n = 0;
while (c >= '0' && c <= '9' )
{
n = n * 10 + (c - '0' );
i++;
c = exp[i];
}
i--;
stack.push(n);
}
else
{
let val1 = stack.pop();
let val2 = stack.pop();
switch (c)
{
case '+' :
stack.push(val2 + val1);
break ;
case '-' :
stack.push(val2 - val1);
break ;
case '/' :
stack.push(parseInt(val2 / val1, 10));
break ;
case '*' :
stack.push(val2 * val1);
break ;
}
}
}
return stack.pop();
}
let exp = "100 200 + 2 / 5 * 7 +" ;
document.write(evaluatePostfix(exp));
</script>
|
Time Complexity: O(N)
Auxiliary Space: O(N)
Feeling lost in the world of random DSA topics, wasting time without progress? It's time for a change! Join our DSA course, where we'll guide you on an exciting journey to master DSA efficiently and on schedule.
Ready to dive in? Explore our Free Demo Content and join our DSA course, trusted by over 100,000 geeks!