Given a string, count the total number of vowels (a, e, i, o, u) in it. There are two methods to count total number of vowels in a string.
- Iterative
- Recursive
Examples:
Input : abc de
Output : 2
Input : geeksforgeeks portal
Output : 7
1. Iterative Method:
Below is the implementation:
C++
#include<iostream>
using namespace std;
bool isVowel( char ch)
{
ch = toupper (ch);
return (ch== 'A' || ch== 'E' || ch== 'I' ||
ch== 'O' || ch== 'U' );
}
int countVowels(string str)
{
int count = 0;
for ( int i=0; i<str.length(); i++)
if (isVowel(str[i]))
++count;
return count;
}
int main()
{
string str = "abc de" ;
cout << countVowels(str) << endl;
return 0;
}
|
Java
public class GFG {
static boolean isVowel( char ch)
{
ch = Character.toUpperCase(ch);
return (ch== 'A' || ch== 'E' || ch== 'I' ||
ch== 'O' || ch== 'U' );
}
static int countVowels(String str)
{
int count = 0 ;
for ( int i = 0 ; i < str.length(); i++)
if (isVowel(str.charAt(i)))
++count;
return count;
}
public static void main(String args[])
{
String str = "abc de" ;
System.out.println(countVowels(str));
}
}
|
Python3
def isVowel(ch):
return ch.upper() in [ 'A' , 'E' , 'I' , 'O' , 'U' ]
def countVowels( str ):
count = 0
for i in range ( len ( str )):
if isVowel( str [i]):
count + = 1
return count
str = 'abc de'
print (countVowels( str ))
|
C#
using System;
class GFG
{
public static bool isVowel( char ch)
{
ch = char .ToUpper(ch);
return (ch == 'A' || ch == 'E' ||
ch == 'I' || ch == 'O' ||
ch == 'U' );
}
public static int countVowels( string str)
{
int count = 0;
for ( int i = 0; i < str.Length; i++)
{
if (isVowel(str[i]))
{
++count;
}
}
return count;
}
public static void Main( string [] args)
{
string str = "abc de" ;
Console.WriteLine(countVowels(str));
}
}
|
PHP
<?php
function isVowel( $ch )
{
$ch = strtoupper ( $ch );
return ( $ch == 'A' || $ch == 'E' ||
$ch == 'I' || $ch == 'O' ||
$ch == 'U' );
}
function countVowels( $str )
{
$count = 0;
for ( $i = 0; $i < strlen ( $str ); $i ++)
if (isVowel( $str [ $i ]))
++ $count ;
return $count ;
}
$str = "abc de" ;
echo countVowels( $str ) . "\n" ;
?>
|
Javascript
<script>
function isVowel(ch) {
ch = ch.toUpperCase();
return ch == "A" || ch == "E" || ch == "I" || ch == "O" || ch == "U" ;
}
function countVowels(str)
{
var count = 0;
for ( var i = 0; i < str.length; i++)
if (isVowel(str[i]))
++count;
return count;
}
var str = "abc de" ;
document.write(countVowels(str));
document.write( "<br>" );
</script>
|
Time Complexity: O(n), where n is the length of the string
Auxiliary Space: O(1)
2. Recursive Method:
Below is the implementation:
C++
#include<iostream>
using namespace std;
bool isVowel( char ch)
{
ch = toupper (ch);
return (ch== 'A' || ch== 'E' || ch== 'I' ||
ch== 'O' || ch== 'U' );
}
int countVovels(string str, int n)
{
if (n == 1)
return isVowel(str[n-1]);
return countVovels(str, n-1) + isVowel(str[n-1]);
}
int main()
{
string str = "abc de" ;
cout << countVovels(str, str.length()) << endl;
return 0;
}
|
Java
public class GFG {
static int isVowel( char ch)
{
ch = Character.toUpperCase(ch);
if (ch== 'A' || ch== 'E' || ch== 'I' ||
ch== 'O' || ch== 'U' )
return 1 ;
else return 0 ;
}
static int countVowels(String str, int n)
{
if (n == 1 )
return isVowel(str.charAt(n - 1 ));
return countVowels(str, n- 1 ) + isVowel(str.charAt(n - 1 ));
}
public static void main(String args[])
{
String str = "abc de" ;
System.out.println(countVowels(str,str.length()));
}
}
|
Python 3
def isVowel(ch):
return ch.upper() in [ 'A' , 'E' , 'I' , 'O' , 'U' ]
def countVovels( str , n):
if (n = = 1 ):
return isVowel( str [n - 1 ]);
return (countVovels( str , n - 1 ) +
isVowel( str [n - 1 ]));
str = "abc de" ;
print (countVovels( str , len ( str )))
|
C#
using System;
public class GFG
{
public static int isVowel( char ch)
{
ch = char .ToUpper(ch);
if (ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U' )
{
return 1;
}
else
{
return 0;
}
}
public static int countVowels( string str, int n)
{
if (n == 1)
{
return isVowel(str[n - 1]);
}
return countVowels(str, n - 1) + isVowel(str[n - 1]);
}
public static void Main( string [] args)
{
string str = "abc de" ;
Console.WriteLine(countVowels(str,str.Length));
}
}
|
PHP
<?php
function isVowel( $ch )
{
$ch = strtoupper ( $ch );
return ( $ch == 'A' || $ch == 'E' ||
$ch == 'I' || $ch == 'O' ||
$ch == 'U' );
}
function countVovels( $str , $n )
{
if ( $n == 1)
return isVowel( $str [ $n - 1]);
return countVovels( $str , $n - 1) +
isVowel( $str [ $n - 1]);
}
$str = "abc de" ;
echo countVovels( $str , strlen ( $str )) . "\n" ;
?>
|
Javascript
<script>
function isVowel(ch)
{
ch = ch.toUpperCase();
return (ch== 'A' || ch== 'E' || ch== 'I' ||
ch== 'O' || ch== 'U' );
}
function countVovels(str,n)
{
if (n == 1)
return isVowel(str[n-1]);
return countVovels(str, n-1) + isVowel(str[n-1]);
}
let str = "abc de" ;
document.write(countVovels(str, str.length));
</script>
|
Time Complexity: O(n), where n is the length of the string
Auxiliary Space: O(n), where n is the length of the string since the function is calling itself n times.
How Recursive Code Working.
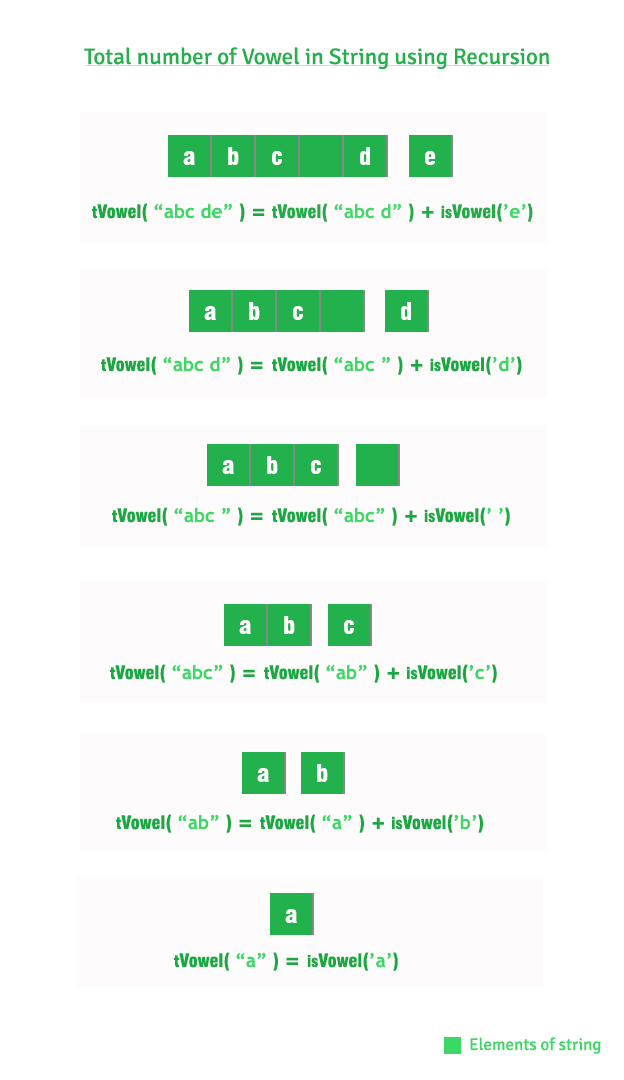
If you like GeeksforGeeks and would like to contribute, you can also write an article using write.geeksforgeeks.org or mail your article to review-team@geeksforgeeks.org. See your article appearing on the GeeksforGeeks main page and help other Geeks.
Feeling lost in the world of random DSA topics, wasting time without progress? It's time for a change! Join our DSA course, where we'll guide you on an exciting journey to master DSA efficiently and on schedule.
Ready to dive in? Explore our Free Demo Content and join our DSA course, trusted by over 100,000 geeks!