Given an array (or string), the task is to reverse the array/string.
Examples :
Input : arr[] = {1, 2, 3}
Output : arr[] = {3, 2, 1}
Input : arr[] = {4, 5, 1, 2}
Output : arr[] = {2, 1, 5, 4}
Iterative way :
1) Initialize start and end indexes as start = 0, end = n-1
2) In a loop, swap arr[start] with arr[end] and change start and end as follows :
start = start +1, end = end – 1
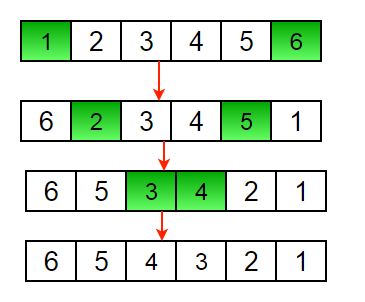
Another example to reverse a string:
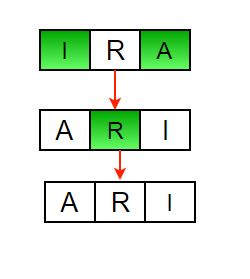
Below is the implementation of the above approach :
C++
#include <bits/stdc++.h>
using namespace std;
void rvereseArray( int arr[], int start, int end)
{
while (start < end)
{
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
void printArray( int arr[], int size)
{
for ( int i = 0; i < size; i++)
cout << arr[i] << " " ;
cout << endl;
}
int main()
{
int arr[] = {1, 2, 3, 4, 5, 6};
int n = sizeof (arr) / sizeof (arr[0]);
printArray(arr, n);
rvereseArray(arr, 0, n-1);
cout << "Reversed array is" << endl;
printArray(arr, n);
return 0;
}
|
C
#include<stdio.h>
void rvereseArray( int arr[], int start, int end)
{
int temp;
while (start < end)
{
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
void printArray( int arr[], int size)
{
int i;
for (i=0; i < size; i++)
printf ( "%d " , arr[i]);
printf ( "\n" );
}
int main()
{
int arr[] = {1, 2, 3, 4, 5, 6};
int n = sizeof (arr) / sizeof (arr[0]);
printArray(arr, n);
rvereseArray(arr, 0, n-1);
printf ( "Reversed array is \n" );
printArray(arr, n);
return 0;
}
|
Java
public class GFG {
static void rvereseArray( int arr[],
int start, int end)
{
int temp;
while (start < end)
{
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
static void printArray( int arr[],
int size)
{
for ( int i = 0 ; i < size; i++)
System.out.print(arr[i] + " " );
System.out.println();
}
public static void main(String args[]) {
int arr[] = { 1 , 2 , 3 , 4 , 5 , 6 };
printArray(arr, 6 );
rvereseArray(arr, 0 , 5 );
System.out.print( "Reversed array is \n" );
printArray(arr, 6 );
}
}
|
Python
def reverseList(A, start, end):
while start < end:
A[start], A[end] = A[end], A[start]
start + = 1
end - = 1
A = [ 1 , 2 , 3 , 4 , 5 , 6 ]
print (A)
reverseList(A, 0 , 5 )
print ( "Reversed list is" )
print (A)
|
C#
using System;
class GFG {
static void rvereseArray( int []arr,
int start, int end)
{
int temp;
while (start < end)
{
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
static void printArray( int []arr,
int size)
{
for ( int i = 0; i < size; i++)
Console.Write(arr[i] + " " );
Console.WriteLine();
}
public static void Main()
{
int []arr = {1, 2, 3, 4, 5, 6};
printArray(arr, 6);
rvereseArray(arr, 0, 5);
Console.Write( "Reversed array is \n" );
printArray(arr, 6);
}
}
|
PHP
<?php
function rvereseArray(& $arr , $start ,
$end )
{
while ( $start < $end )
{
$temp = $arr [ $start ];
$arr [ $start ] = $arr [ $end ];
$arr [ $end ] = $temp ;
$start ++;
$end --;
}
}
function printArray(& $arr , $size )
{
for ( $i = 0; $i < $size ; $i ++)
echo $arr [ $i ] . " " ;
echo "\n" ;
}
$arr = array (1, 2, 3, 4, 5, 6);
printArray( $arr , 6);
rvereseArray( $arr , 0, 5);
echo "Reversed array is" . "\n" ;
printArray( $arr , 6);
?>
|
javascript
function rvereseArray(arr,start,end)
{
while (start < end)
{
var temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
start++;
end--;
}
}
function printArray(arr,size)
{
for ( var i = 0; i < size; i++){
console.log(arr[i]);
}
}
var arr= [1, 2, 3, 4, 5, 6];
var n = 6;
printArray(arr, n);
rvereseArray(arr, 0, n-1);
console.log( "Reversed array is" );
printArray(arr, n);
|
Output :
1 2 3 4 5 6
Reversed array is
6 5 4 3 2 1
Time Complexity: O(n)
Auxiliary Space: O(1)
Recursive Way :
1) Initialize start and end indexes as start = 0, end = n-1
2) Swap arr[start] with arr[end]
3) Recursively call reverse for rest of the array.
Below is the implementation of the above approach :
C++
#include <bits/stdc++.h>
using namespace std;
void rvereseArray( int arr[], int start, int end)
{
if (start >= end)
return ;
int temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
rvereseArray(arr, start + 1, end - 1);
}
void printArray( int arr[], int size)
{
for ( int i = 0; i < size; i++)
cout << arr[i] << " " ;
cout << endl;
}
int main()
{
int arr[] = {1, 2, 3, 4, 5, 6};
printArray(arr, 6);
rvereseArray(arr, 0, 5);
cout << "Reversed array is" << endl;
printArray(arr, 6);
return 0;
}
|
C
#include <stdio.h>
void rvereseArray( int arr[], int start, int end)
{
int temp;
if (start >= end)
return ;
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
rvereseArray(arr, start+1, end-1);
}
void printArray( int arr[], int size)
{
int i;
for (i=0; i < size; i++)
printf ( "%d " , arr[i]);
printf ( "\n" );
}
int main()
{
int arr[] = {1, 2, 3, 4, 5, 6};
printArray(arr, 6);
rvereseArray(arr, 0, 5);
printf ( "Reversed array is \n" );
printArray(arr, 6);
return 0;
}
|
Java
import java.io.*;
class ReverseArray {
static void rvereseArray( int arr[], int start, int end)
{
int temp;
if (start >= end)
return ;
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
rvereseArray(arr, start+ 1 , end- 1 );
}
static void printArray( int arr[], int size)
{
for ( int i= 0 ; i < size; i++)
System.out.print(arr[i] + " " );
System.out.println( "" );
}
public static void main (String[] args) {
int arr[] = { 1 , 2 , 3 , 4 , 5 , 6 };
printArray(arr, 6 );
rvereseArray(arr, 0 , 5 );
System.out.println( "Reversed array is " );
printArray(arr, 6 );
}
}
|
Python
def reverseList(A, start, end):
if start > = end:
return
A[start], A[end] = A[end], A[start]
reverseList(A, start + 1 , end - 1 )
A = [ 1 , 2 , 3 , 4 , 5 , 6 ]
print (A)
reverseList(A, 0 , 5 )
print ( "Reversed list is" )
print (A)
|
C#
using System;
class GFG
{
static void rvereseArray( int []arr, int start,
int end)
{
int temp;
if (start >= end)
return ;
temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
rvereseArray(arr, start+1, end-1);
}
static void printArray( int []arr, int size)
{
for ( int i = 0; i < size; i++)
Console.Write(arr[i] + " " );
Console.WriteLine( "" );
}
public static void Main ()
{
int []arr = {1, 2, 3, 4, 5, 6};
printArray(arr, 6);
rvereseArray(arr, 0, 5);
Console.WriteLine( "Reversed array is " );
printArray(arr, 6);
}
}
|
PHP
<?php
function rvereseArray(& $arr ,
$start , $end )
{
if ( $start >= $end )
return ;
$temp = $arr [ $start ];
$arr [ $start ] = $arr [ $end ];
$arr [ $end ] = $temp ;
rvereseArray( $arr , $start + 1,
$end - 1);
}
function printArray(& $arr , $size )
{
for ( $i = 0; $i < $size ; $i ++)
echo $arr [ $i ] . " " ;
echo "\n" ;
}
$arr = array (1, 2, 3, 4, 5, 6);
printArray( $arr , 6);
rvereseArray( $arr , 0, 5);
echo "Reversed array is" . "\n" ;
printArray( $arr , 6);
?>
|
javascript
function rvereseArray(arr,start,end)
{
var temp = arr[start];
arr[start] = arr[end];
arr[end] = temp;
if (start+1<end-1){
rvereseArray(arr, start + 1, end - 1);
}
}
function printArray(arr,size)
{
for ( var i = 0; i < size; i++){
console.log(arr[i]);
}
}
var arr= [1, 2, 3, 4, 5, 6];
printArray(arr, 6);
rvereseArray(arr, 0, 5);
console.log( "Reversed array is" );
printArray(arr, 6);
|
Output :
1 2 3 4 5 6
Reversed array is
6 5 4 3 2 1
Time Complexity: O(n)
Auxiliary Space: O(n)
Please write comments if you find any bug in the above programs or other ways to solve the same problem.
Feeling lost in the world of random DSA topics, wasting time without progress? It's time for a change! Join our DSA course, where we'll guide you on an exciting journey to master DSA efficiently and on schedule.
Ready to dive in? Explore our Free Demo Content and join our DSA course, trusted by over 100,000 geeks!